Resumo : neste tutorial, você aprenderá como herdar da ttk.Frame
classe e usá-la na janela raiz.
No tutorial anterior , você aprendeu como subclassificar a Tkinter.Tk
classe. Entretanto, uma aplicação Tkinter deve ter apenas uma Tk
instância.
Portanto, é comum herdar da ttk.Frame
classe e usar a subclasse na janela raiz.
Para herdar a ttk.Frame
classe, você usa a seguinte sintaxe:
class MainFrame(ttk.Frame):
pass
Linguagem de código: Python ( python )
Como a Frame
precisa de um contêiner, você precisa adicionar um argumento ao seu __init__()
método e chamar o __init__()
método da ttk.Frame
classe assim:
class MainFrame(ttk.Frame):
def __init__(self, container):
super().__init__(container)
Linguagem de código: Python ( python )
O seguinte mostra a classe completa MainFrame
que possui um rótulo e um botão . Quando você clica no botão, ele mostra uma caixa de mensagem :
class MainFrame(ttk.Frame):
def __init__(self, container):
super().__init__(container)
options = {'padx': 5, 'pady': 5}
# label
self.label = ttk.Label(self, text='Hello, Tkinter!')
self.label.pack(**options)
# button
self.button = ttk.Button(self, text='Click Me')
self.button['command'] = self.button_clicked
self.button.pack(**options)
# show the frame on the container
self.pack(**options)
def button_clicked(self):
showinfo(title='Information',
message='Hello, Tkinter!')
Linguagem de código: Python ( python )
O seguinte define uma App
classe que herda da Tk
classe:
class App(tk.Tk):
def __init__(self):
super().__init__()
# configure the root window
self.title('My Awesome App')
self.geometry('300x100')
Linguagem de código: Python ( python )
E você pode inicializar o aplicativo por meio do if __name__ == "__main__"
bloco.
if __name__ == "__main__":
app = App()
frame = MainFrame(app)
app.mainloop()
Linguagem de código: Python ( python )
Neste código:
- Primeiro, crie uma nova instância da
App
classe. - Segundo, crie uma nova instância da
MainFrame
classe e defina seu contêiner para a instância do aplicativo. - Terceiro, inicie o aplicativo chamando app(). Ele executará o
__call__()
método que invocará amainloop()
janela raiz.
Junte tudo:
import tkinter as tk
from tkinter import ttk
from tkinter.messagebox import showinfo
class MainFrame(ttk.Frame):
def __init__(self, container):
super().__init__(container)
options = {'padx': 5, 'pady': 5}
# label
self.label = ttk.Label(self, text='Hello, Tkinter!')
self.label.pack(**options)
# button
self.button = ttk.Button(self, text='Click Me')
self.button['command'] = self.button_clicked
self.button.pack(**options)
# show the frame on the container
self.pack(**options)
def button_clicked(self):
showinfo(title='Information',
message='Hello, Tkinter!')
class App(tk.Tk):
def __init__(self):
super().__init__()
# configure the root window
self.title('My Awesome App')
self.geometry('300x100')
if __name__ == "__main__":
app = App()
frame = MainFrame(app)
app.mainloop()
Linguagem de código: Python ( python )
Saída:
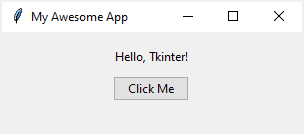
Mais exemplo de quadro orientado a objetos
O exemplo a seguir usa as classes para converter a janela Substituir do Frame
tutorial :
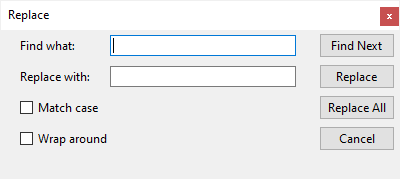
import tkinter as tk
from tkinter import ttk
class InputFrame(ttk.Frame):
def __init__(self, container):
super().__init__(container)
# setup the grid layout manager
self.columnconfigure(0, weight=1)
self.columnconfigure(0, weight=3)
self.__create_widgets()
def __create_widgets(self):
# Find what
ttk.Label(self, text='Find what:').grid(column=0, row=0, sticky=tk.W)
keyword = ttk.Entry(self, width=30)
keyword.focus()
keyword.grid(column=1, row=0, sticky=tk.W)
# Replace with:
ttk.Label(self, text='Replace with:').grid(
column=0, row=1, sticky=tk.W)
replacement = ttk.Entry(self, width=30)
replacement.grid(column=1, row=1, sticky=tk.W)
# Match Case checkbox
match_case = tk.StringVar()
match_case_check = ttk.Checkbutton(
self,
text='Match case',
variable=match_case,
command=lambda: print(match_case.get()))
match_case_check.grid(column=0, row=2, sticky=tk.W)
# Wrap Around checkbox
wrap_around = tk.StringVar()
wrap_around_check = ttk.Checkbutton(
self,
variable=wrap_around,
text='Wrap around',
command=lambda: print(wrap_around.get()))
wrap_around_check.grid(column=0, row=3, sticky=tk.W)
for widget in self.winfo_children():
widget.grid(padx=0, pady=5)
class ButtonFrame(ttk.Frame):
def __init__(self, container):
super().__init__(container)
# setup the grid layout manager
self.columnconfigure(0, weight=1)
self.__create_widgets()
def __create_widgets(self):
ttk.Button(self, text='Find Next').grid(column=0, row=0)
ttk.Button(self, text='Replace').grid(column=0, row=1)
ttk.Button(self, text='Replace All').grid(column=0, row=2)
ttk.Button(self, text='Cancel').grid(column=0, row=3)
for widget in self.winfo_children():
widget.grid(padx=0, pady=3)
class App(tk.Tk):
def __init__(self):
super().__init__()
self.title('Replace')
self.geometry('400x150')
self.resizable(0, 0)
# windows only (remove the minimize/maximize button)
self.attributes('-toolwindow', True)
# layout on the root window
self.columnconfigure(0, weight=4)
self.columnconfigure(1, weight=1)
self.__create_widgets()
def __create_widgets(self):
# create the input frame
input_frame = InputFrame(self)
input_frame.grid(column=0, row=0)
# create the button frame
button_frame = ButtonFrame(self)
button_frame.grid(column=1, row=0)
if __name__ == "__main__":
app = App()
app.mainloop()
Linguagem de código: Python ( python )
Resumo
- Subclasse
ttk.Frame
e inicialize os widgets no quadro. - Use a subclasse de
ttk.Frame
em uma janela raiz.